How to accept crypto payments on any e-commerce store
Step-1: Login / create an account on intrXn by visiting https://business.intrxn.com
Go to API integration tab -> Click on API integration
Provide ecommerce / website link
Add webhook callback url in the same domain name
Press the generate button to generate API & secret key
Step-2: Merchant will be given a specific API end point to post the order information.
Step-3: When posting to the intrXn API endpoint with order information, the merchant has to pass:
- Total amount
- Currency Format (which will default to his overall settings)
- Order Id (should be unique)
- Callback url – which we will redirect after invoice payment.
Step-4: And send the above request with 2 custom headers as follows:
- API Key Header
- Timestamp Header
Example:
- X-intrXn-key <api-key>
- X-intrXn-Timestamp<Timestamp>
intrXn app will verify the api request by validating the api-key and if it matches, processes the request
/** header */
var myHeaders = new Headers();
myHeaders.append("X-intrXn-key", "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx");
// API-Key
myHeaders.append("X-intrXn-Timestamp", 234234243234); // timestamp
myHeaders.append("Content-Type", "application/json");
/** body */ {
"orderId": String,
"amount": Integer,
"currency": String, // format code
"redirectUrl": String
}
/** Sample code */
const timestamp = Math.floor(new Date() / 1000);
var myHeaders = new Headers();
myHeaders.append("X-intrXn-key",
"xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"); // API-Key
myHeaders.append("X-intrXn-Timestamp", timestamp);
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"orderId":"1234",
"amount":100,
"currency": "AED",
"redirectUrl":"http://www.test.com/"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("http://localhost:3001/v1/payment", requestOptions)
.then(response => response.json())
.then(result => {
if(result.result.status) window.location=result.result.
redirectUrl;
if(!result.result.status) alert(result.result.message);
})
.catch(error => console.log('error', error));
intrXn Background Process:
intrxn will schedule tasks which runs at a given frequency will verify the crypto invoice payments and on success or failure, will call the webhook url (provided by the merchants) with payment status info and sign the request with specific merchant key and pass it as a header.
Example:
X-intrXn-wh-signature <HMAC signature>
router.post('/webhook', async (req, res, next) => {
const { paymentStatus, orderId, paymentId } = req.body;
cosnt hash = req.headers["x-intrxn-wh-signature"]
const secretKey = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"; // SecretKey
const newHash = crypto
.createHmac("sha256", secretKey)
.update(JSON.stringify(data), "utf-8")
.digest("hex");
if(hash === newHash) {
// do something
return { status: true }
}
return { status: false }
});
** Expected response from webhook URL status - true or false
/** body */
{
paymentStatus: "SUCCESS", // SUCCESS or FAILED
orderId: 'xxxxxxxxxx',
paymentId: 'xxxxxxxxxxx'
}
/** Response */
{
status: false // true or false
}
Merchant Background Process
Ecommerce backend will validate the signature and if it passes, should process our api call and process further. (Example – They can update their database and send a confirmation mail to the customers about order being accepted and further process start message)
If the payment status is failed for some reason, intrXn will notify the same to ecommerce portal but the refund will be a manual process.
Blockroute Technologies LLC
Office no LX 2202, API Tower – Shaikh Zayed Road – Dubai
- hello@intrxn.com
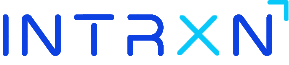
© Copyright 2019 Blockroute Technologies LLC. All right reserved.